Getting started with first Ruby on Rails app
Create the basic Ruby on Rails application and understand the CRUD concept
Now, we are ready to create our first Ruby on Rails application.
Ruby on Rails Installation
Install the Ruby on Rails framework by running the following command:
gem install rails
This will install the latest version of Rails.
Try verifying by checking the version as:
rails -v
# output : Rails 7.0.4.3
Getting started with app
We will be creating a Pasal(or store) for storing ours ideas. We should be able to create, view, update and delete our idea in this pasal.
Go through the following commands to get started with application:
-
Let’s start with creating the application by running following command:
rails new ideapasal
-
Enter into the folder
ideapasal
and open the application in the editor:cd ideapasal code .
NOTE: You can use different editor as well other than VS Code.
-
Now run the server by running following command:
rails s
-
Open the url http://localhost:3000 in the browser, you should see following output:
Create the scaffold for Idea
Now, we are going to Model, Views and Controller to manage our idea in the pasal.
-
Run the following command to create the scaffold of idea that will allows you to list, add, remove, edit, and view ideas:
rails generate scaffold idea name:string description:text image:string
-
Run the following command to update the database and restart the server:
rails db:migrate rails server
-
Open http://localhost:3000/ideas in your Browser to view the list of ideas.
-
Now, try to create a new idea and view it. Also, try to update existing idea and finally try to delete them.
Update the root route of the application
Currently, our main route i.e /
is displaying the default Rails application page.
Let’s try to display the ideas which we saw earlier in /ideas
route.
Open the config/routes.rb
file. After the first line, add this line and save it:
root to: 'ideas#index'
The final config/routes.rb
fill will look like below:
Rails.application.routes.draw do
root to: 'ideas#index'
resources :ideas
end
NOTE: You should put the root route at the top of the file, because it is the most popular route and should be matched first.
Now, try to open http://localhost:3000/ in your Browser. It will now show the list of ideas.
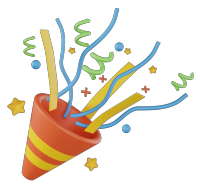
Congratulations!
You have now created your first application in Ruby on Rails.Click Next to proceed to styling the application.
Help me to improve Dhanu Sir.